Concurrency in Java: Best Practices for Multithreaded Programming
- raginijhaq121
- Jun 27, 2024
- 4 min read
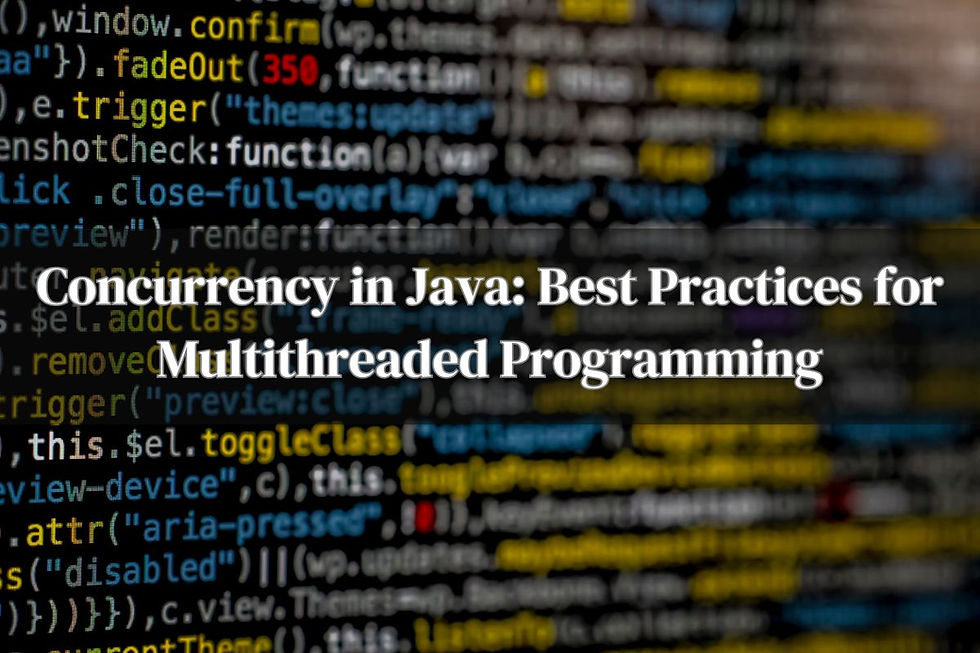
Introduction
Concurrency in Java refers to the ability of a program to execute multiple tasks simultaneously, utilizing the capabilities of modern multicore processors effectively. Java's support for multithreading allows developers to create applications that can perform multiple tasks concurrently, enhancing performance and responsiveness. However, concurrent programming introduces challenges such as thread safety, deadlock, and race conditions, which must be carefully managed to ensure the reliability and correctness of the software.
Understanding Concurrency in Java
Concurrency in Java is primarily achieved through the Thread class and the Runnable interface, which enable the creation and execution of multiple threads within a Java program. Threads represent independent paths of execution that can run concurrently with other threads, allowing developers to parallelize tasks and improve application performance.
Best Practices for Multithreaded Programming
Use Executors from java.util.concurrent: Instead of directly managing threads using Thread objects, utilize executors and thread pools from the java.util.concurrent package. Executors provide a higher-level abstraction for managing threads, handling tasks, and scheduling execution, which simplifies concurrency management and improves scalability.
Thread Safety with Synchronization: Ensure that shared data accessed by multiple threads is synchronized properly to prevent race conditions. Use synchronized blocks or methods, or employ thread-safe data structures such as ConcurrentHashMap or Atomic classes to manage shared state safely across threads.
Avoid Blocking Operations: Minimize the use of blocking operations within critical sections of code. Long-running or blocking operations can lead to thread contention and reduce the efficiency of concurrency. Consider asynchronous programming techniques using CompletableFuture or reactive programming frameworks to handle non-blocking operations effectively.
Use volatile Keyword for Visibility: Use the volatile keyword to ensure visibility of shared variables across threads. volatile variables are not cached locally by threads and reflect the most up-to-date value, ensuring consistent behavior when accessed by multiple threads without the need for explicit synchronization.
Avoid Deadlock Conditions: Deadlock occurs when two or more threads are blocked indefinitely, waiting for each other to release resources. To prevent deadlock, acquire locks in a consistent order, use timeouts with locks, and minimize the scope of synchronized blocks to reduce the risk of circular dependencies between threads.
Graceful Thread Termination: Ensure threads terminate gracefully when they are no longer needed. Use interruption mechanisms (Thread.interrupt()), ExecutorService.shutdown() methods, and appropriate shutdown hooks to clean up resources and release thread resources properly, preventing resource leaks and improving application stability.
Monitor and Tune Thread Usage: Monitor thread utilization and performance using tools like Java Management Extensions (JMX) or profilers. Analyze thread dumps to identify bottlenecks, excessive thread creation, or thread contention issues, and tune thread pool sizes and configurations based on application workload and hardware characteristics.
Adoption of Modern Java Features
Java continues to evolve with each new release, introducing features that enhance the management of concurrency and improve developer productivity. Features such as the CompletableFuture API for asynchronous programming, enhancements to the java.util.concurrent package, and the introduction of reactive programming libraries like Project Reactor enable developers to tackle complex concurrency challenges more effectively.
Handling Asynchronous Operations
In modern Java development, handling asynchronous operations is crucial for building responsive and scalable applications. The CompletableFuture API simplifies asynchronous programming by providing a fluent interface to compose asynchronous computations, handle dependencies between tasks, and manage exceptions asynchronously. This approach avoids blocking threads and maximizes resource utilization, improving overall application performance.
Scalability and Distributed Computing
With the rise of cloud computing and distributed systems, scalability becomes a primary concern for Java developers. Frameworks like Akka and libraries such as Apache Kafka facilitate scalable, distributed computing by providing actor-based concurrency models, event-driven architectures, and reliable messaging systems. These tools enable developers to build resilient, highly available systems that can handle large-scale concurrent workloads efficiently.
Continued Learning and Adaptation
Effective concurrency management in Java requires continuous learning and adaptation to new tools, techniques, and best practices. Developers should stay updated with the latest advancements in Java concurrency frameworks, performance tuning strategies, and monitoring tools to optimize application performance, identify bottlenecks, and ensure reliable operation in production environments.
Challenges and Considerations
While Java provides powerful tools and frameworks for managing concurrency, developers must navigate several challenges to ensure the reliability and performance of multithreaded applications:
Complexity: Concurrent programming introduces complexities such as race conditions, deadlock, and thread starvation. Understanding these issues and applying appropriate synchronization mechanisms is crucial to maintaining application correctness.
Performance Overhead: Synchronization mechanisms and thread coordination incur performance overhead. Developers must carefully design and optimize concurrent algorithms to minimize contention and maximize throughput.
Scalability: Scalability concerns arise when scaling multithreaded applications across multiple cores or distributed environments. Effective thread pool management, load balancing strategies, and distributed computing frameworks are essential for achieving scalable concurrency.
Emerging Trends and Future Directions
Looking ahead, several trends and advancements are shaping the future of concurrency in Java:
Reactive Programming: Reactive programming paradigms such as Reactive Streams and frameworks like Project Reactor enable developers to build responsive, event-driven applications that efficiently handle asynchronous and concurrent operations.
Actor Model: Actor-based concurrency models, popularized by frameworks like Akka, provide lightweight, isolated units of execution (actors) that communicate via message passing. This approach simplifies concurrent programming by reducing shared mutable state and improving fault tolerance.
Machine Learning and Big Data: The increasing adoption of machine learning algorithms and big data processing frameworks (e.g., Apache Spark) necessitates efficient concurrent data processing and distributed computing capabilities in Java applications.
Conclusion:
Concurrency remains a cornerstone of modern Java development, enabling developers to harness the full potential of multicore processors and distributed computing environments. By embracing best practices, leveraging advanced Java features and frameworks, and staying informed about emerging trends, developers can build resilient, scalable, and high-performance applications that meet the demands of today's dynamic computing landscape.
In conclusion, Java's robust concurrency model, coupled with continuous learning and adaptation to new technologies, empowers developers to tackle complex challenges and innovate with confidence in multithreaded programming. As Java evolves and new paradigms emerge, mastering concurrency will remain essential for building competitive and efficient software solutions.
For developers looking to deepen their understanding of Java concurrency and stay ahead in the rapidly evolving field of software development, investing in quality Best Java training in Ahmedabad, Nashik, Gurgson, Delhi and other cities in India can provide invaluable insights and hands-on experience.
Comments