Harnessing the Power of Python Generators and Iterators
- raginijhaq121
- Jun 18, 2024
- 4 min read
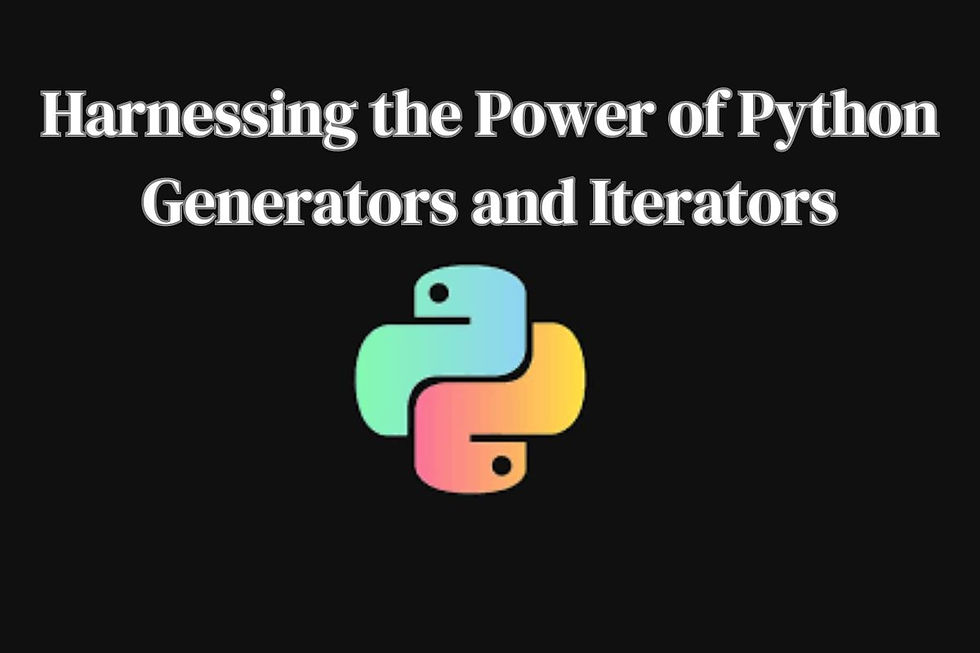
Introduction:
In Python programming, dealing with large datasets and streams of data efficiently is essential. Generators and iterators are powerful tools that help achieve this efficiency by conserving memory and enhancing performance. Understanding and utilizing these tools can significantly improve your coding skills and the effectiveness of your applications.
What Are Iterators?
An iterator in Python is an object that enables traversal through a container, such as a list or a dictionary. It implements two essential methods: iter() and next(). The iter() method initializes the iterator and returns the iterator object itself, while the next() method returns the next item from the container. When there are no more items to return, it raises a StopIteration exception.
What Are Generators?
Generators simplify the creation of iterators through the use of a function rather than a class. By using the yield keyword, generators produce items one at a time and maintain their state between each yield. This approach, known as lazy evaluation, means that generators generate items only when needed, making them highly memory-efficient.
Advantages of Using Generators
Memory Efficiency: Generators don't store the entire dataset in memory; they generate items on-the-fly. This is particularly beneficial for processing large datasets.
Performance: Generators often perform faster than functions that build and return lists because they yield values as they are produced.
Simplicity: The use of the yield keyword makes the code more readable and concise, eliminating the need for an explicit iterator class.
Pipeline Design: Generators can be chained together to form a pipeline, processing data in stages efficiently.
Practical Applications of Generators and Iterators
Reading Large Files: When dealing with large files, reading the entire file into memory is impractical. Generators allow processing the file line by line, thus conserving memory.
Streaming Data: In real-time data processing scenarios, such as reading data from a sensor or a web service, generators provide a way to handle continuous data streams without loading all data into memory.
Infinite Sequences: Generators can represent infinite sequences, such as the Fibonacci series or an endless sequence of numbers, by yielding values indefinitely.
Combining Generators and Iterators
Generators and iterators can be combined to create complex data processing pipelines. For example, consider a scenario where you need to read data from a file, filter out specific information, and then transform the data. Using generators, you can build a pipeline that reads the data, filters lines containing a specific keyword, and then transforms these lines, all while maintaining memory efficiency and readability.
Deep Dive into Generators and Iterators
Iterators: A Closer Look
To fully appreciate the power of iterators, it's essential to understand their underlying mechanics. An iterator object in Python must implement two specific methods:
iter(): This method initializes the iterator. It is called at the start of an iteration and should return the iterator object itself.
next(): This method retrieves the next item in the sequence. It returns the next value and advances the iterator. If there are no more items to return, it raises a StopIteration exception, signaling the end of the iteration.
Iterators are particularly useful for creating custom data structures that can be traversed using a loop, providing more control over the iteration process compared to built-in iterable objects like lists or tuples.
Generators: Beyond Basics
Generators extend the capabilities of iterators by simplifying their creation. A generator is essentially a function that uses the yield keyword to return values one at a time, suspending its state between each yield. This means that the function can produce a series of values over time, rather than computing them all at once and sending them back in a list.
Here are a few key points about generators:
State Retention: Each time a generator's yield statement is executed, the function's state (including local variables and the current execution point) is saved. When the generator is called again, it resumes execution from where it left off.
Automatic StopIteration: Generators automatically raise a Stop Iteration exception when they exhaust their items. This makes them seamlessly integrate with loops and other iteration constructs in Python.
Single-use: Generators can be iterated over only once. After all items have been yielded, the generator cannot be restarted or reused.
Practical Applications
Let's explore a few more practical applications where generators and iterators can significantly enhance performance and readability.
Data Transformation Pipelines: Generators can be used to create data transformation pipelines that process data in a series of steps, each step represented by a generator. This approach is efficient and modular, allowing each step to be tested and debugged independently.
Resource Management: Generators can be used to manage resources such as file handles or network connections, ensuring they are opened only when needed and properly closed afterward. This is particularly useful in scenarios requiring robust error handling and resource cleanup.
Complex Iterations: Iterators can be used to create complex iteration patterns over custom data structures, such as trees, graphs, or nested collections. By implementing custom iteration logic, developers can traverse these structures in a controlled and efficient manner.
Advanced Patterns with Generators
Generator Expressions: Similar to list comprehensions, generator expressions provide a concise way to create generators. They are written using a syntax similar to list comprehensions but with parentheses instead of square brackets. This makes them an excellent choice for creating simple, on-the-fly generators.
Coroutines: Generators can also be used as coroutines, allowing for more complex asynchronous programming. By using the yield keyword to produce values and also to receive values sent into the generator, coroutines can maintain state across multiple entry points, enabling sophisticated control flows and state management.
Conclusion:
Generators and iterators are fundamental concepts in Python that empower developers to write efficient, scalable, and maintainable code. By leveraging these tools effectively, Python programmers can handle diverse data processing challenges with ease, from managing extensive datasets to orchestrating complex workflows.
Understanding the nuances of generators and iterators not only enhances performance but also fosters a deeper appreciation for Python's capabilities in handling data-centric applications. Whether you're enrolled in a Python course in Ahmedabad, Nashik, Delhi and other cities in India or developing applications globally, mastering generators and iterators will undoubtedly elevate your programming skills and enable you to tackle advanced programming tasks with confidence.
Comments